Generic(< >)
- 배열의 타입을 지정해줌
- 클래스타입을 generic에 넣어주거나, 선언 시 특정 타입을 지정해주지 않으면 객체화시 마다 타입변경 가능
- 클래스를 타입으로 넣어줄 경우 한번에 여러 값을 넣어줄 수 있음
- ex) 타입이 <BoardBean>인 ArrayList 안에 있는 모든 멤버는 BoardBean타입임
객체화 시 <>안에 값이 바뀔 때마다 변경됨
- list.jsp(게시판 만들기)
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="https://code.jquery.com/jquery-3.6.3.min.js"></script>
<style>
table, th, td{
border: 1px solid black;
border-collapse: collapse;
padding: 5px 10px;
text-align: center;
}
button{
margin: 5px;
}
</style>
</head>
<body>
<button onclick="location.href='writeForm.jsp'">글쓰기</button>
<table>
<colgroup>
<col width="10%"/>
<col width="50%"/>
<col width="20%"/>
<col width="20%"/>
</colgroup>
<thead>
<tr>
<th>no</th>
<th>제목</th>
<th>작성자</th>
<th>삭제</th>
</tr>
</thead>
<tbody>
<c:if test="${list.size() == 0}">
<tr>
<th colspan="4">작성된 글이 존재하지 않습니다.</th>
</tr>
</c:if>
<!-- el태그에서는 객체 내 private 필드를 getter()를 사용하지 않고 꺼내올 수 있음 -->
<c:if test="${list.size() > 0}">
<c:forEach items="${list}" var="board" varStatus="stat">
<tr>
<td>${stat.index}</td>
<td><a href="detail?idx=${stat.index}">${board.subject}</a></td>
<td>${board.user_name}</td>
<td><a href="remove?idx=${stat.index}">삭제</a></td>
</tr>
</c:forEach>
</c:if>
</tbody>
</table>
</body>
<script></script>
</html>
# el태그(${})에서는 객체 내 private 필드를 getter()를 사용하지 않고 꺼내올 수 있음
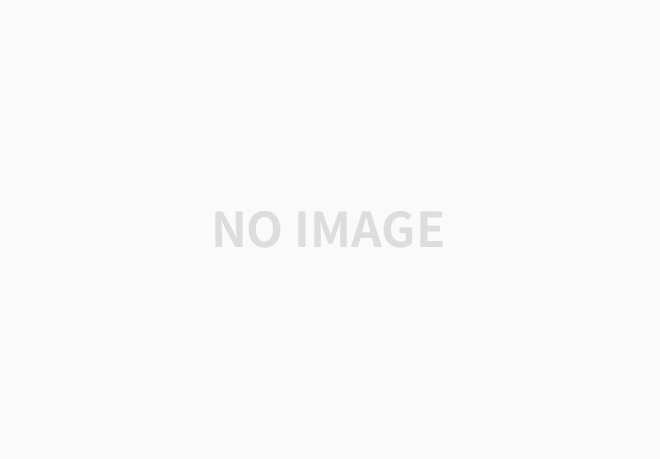
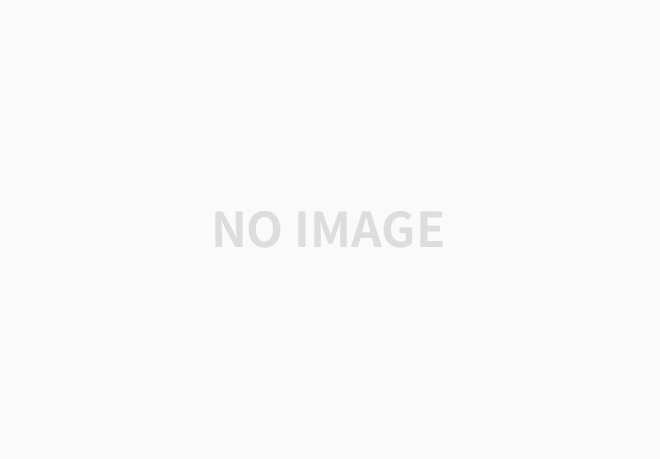
- BoardController
package kr.co.web.controller;
import java.io.IOException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import kr.co.web.model.BoardModel;
@WebServlet(urlPatterns = {"/","/write","/detail","/remove"})
public class BoardController extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
dual(req,resp);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
dual(req,resp);
}
private void dual(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
req.setCharacterEncoding("UTF-8");
String uri = req.getRequestURI(); // /23_board/writeForm.jsp
String ctx = req.getContextPath(); // /23_board
String addr = uri.substring(ctx.length()); // /writeForm.jsp
//substring : uri값에서 ctx.length의 길이 다음부터 끝까지의 값을 가져옴
//System.out.println(addr);
RequestDispatcher dis;
BoardModel model = new BoardModel();
if(addr.equals("/")) {
req.setAttribute("list", model.getList());
dis = req.getRequestDispatcher("list.jsp");
dis.forward(req, resp);
}
if(addr.equals("/write")) {
String user_name = req.getParameter("user_name");
String subject = req.getParameter("subject");
String content = req.getParameter("content");
//System.out.println(user_name+" / "+subject+" / "+content);
model.write(user_name, subject, content);
resp.sendRedirect("list.jsp");
}
if(addr.equals("/detail")) {
String idx = req.getParameter("idx");
//System.out.println("detail : " + idx);
req.setAttribute("board", model.detail(idx));
dis = req.getRequestDispatcher("detail.jsp");
dis.forward(req, resp);
}
if(addr.equals("/remove")) {
String idx = req.getParameter("idx");
//System.out.println("remove : " + idx);
model.remove(idx);
resp.sendRedirect(ctx);
}
}
}
- BoardModel
package kr.co.web.model;
import java.util.ArrayList;
public class BoardModel {
//user_name, subject, content
//어떤 데이터 타입을 사용해야 하나의 리스트에 3개 값이 들어갈까? : class(beans)
private static ArrayList<BoardBean> list = new ArrayList<BoardBean>();
//글쓰기
public void write(String user_name, String subject, String content) {
//1.객체를 생성해서
BoardBean bean = new BoardBean();
//2.받아온 값을 넣고
bean.setUser_name(user_name);
bean.setSubject(subject);
bean.setContent(content);
//3.해당 객체를 리스트에 넣음
list.add(bean);
}
//리스트 불러오기
public ArrayList<BoardBean> getList(){
return list;
}
public BoardBean detail(String idx) {
return list.get(Integer.parseInt(idx));
}
public void remove(String idx) {
list.remove(Integer.parseInt(idx));
}
}
- BoardBean
package kr.co.web.model;
//bean규약 : private으로 생성하고 getter와 setter로 다룸
public class BoardBean {
private String user_name;
private String subject;
private String content;
public String getUser_name() {
return user_name;
}
public void setUser_name(String user_name) {
this.user_name = user_name;
}
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
}
- Box(generic 사용 예시)
package kr.co.web.model;
//클래스의 멤버들은 한번 데이터 타입이 지정되면 변경할 수 없음
//generic으로 가능하게 할 수 있음
//generic을 사용하면 클래스를 객체화하면서 멤버들의 데이터 타입을 유연하게 지정할 수 있음
public class Box<O,N,G> { //해당 클래스는 객체화할 때 멤버들이 <>안에 데이터 타입을 따라감
public O no;
public N name;
public G grade;
}
//Box 클래스는 생성시 타입을 지정해주지 않아 객체화시마다 변경가능
Box box = new Box();
box.no = 13;
box.name = "사과박스";
box.grade = 'A';
//박스에 숫자만 넣고 싶다면?
Box<Integer> box = new Box<Integer>();
box.no = 13;
box.name = 12345;
box.grade = 1;
//문자열, 숫자, 문자열
Box<String, Integer, String> box = new Box<String, Integer, String>();
box.no = "1";
box.name = 1234;
box.grade = "A";
- writerForm.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="https://code.jquery.com/jquery-3.6.3.min.js"></script>
<style>
table, th, td{
border: 1px solid black;
border-collapse: collapse;
padding: 5px 10px;
/* text-align: center; */
}
button{
margin: 5px;
}
table{
width: 500px;
}
input[type="text"]{
width:100%;
}
textarea{
width: 100%;
height: 150px;
resize: none;
}
</style>
</head>
<body>
<form action="write" method="post">
<table>
<tr>
<th>작성자</th>
<td><input type="text" name="user_name"/></td>
</tr>
<tr>
<th>제목</th>
<td><input type="text" name="subject"/></td>
</tr>
<tr>
<th>내용</th>
<td><textarea name="content"></textarea></td>
</tr>
<tr>
<th colspan="2"><button>작성</button></th>
</tr>
</table>
</form>
</body>
<script></script>
</html>
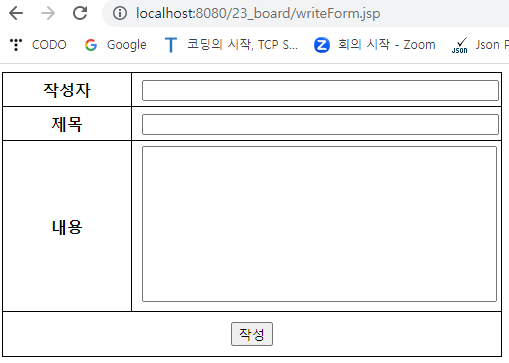
- detail.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="https://code.jquery.com/jquery-3.6.3.min.js"></script>
<style>
table, th, td{
border: 1px solid black;
border-collapse: collapse;
padding: 5px 10px;
/* text-align: center; */
}
table{
width: 500px;
}
</style>
</head>
<body>
<table>
<colgroup>
<col width="20%"/>
<col width="80%"/>
</colgroup>
<tr>
<th>작성자</th>
<td>${board.user_name}</td>
</tr>
<tr>
<th>제목</th>
<td>${board.subject}</td>
</tr>
<tr>
<th>내용</th>
<td>${board.content}</td>
</tr>
<tr>
<th colspan="2"><a href="./">LIST로 돌아가기</a></th>
</tr>
</table>
</body>
<script></script>
</html>

'코딩도전기 > MVC' 카테고리의 다른 글
CODO Day26_JAVA_MVC(Map – Hash Map) (2) | 2023.03.09 |
---|---|
CODO Day25_JAVA_MVC(Linked List/Set Collection(HashSet)) (0) | 2023.03.08 |
CODO Day23~24_JAVA_MVC(Collection Framework - ArrayList/Vector) (0) | 2023.03.06 |
CODO Day23_JAVA_MVC(Exception) (0) | 2023.03.06 |
CODO Day22_JAVA_MVC(String) (0) | 2023.03.06 |